In this article, I will show you how to use a HC-SR04 ultrasonic sensor to measure distance between your sensor and an object in its way using Raspberry Pi. Let's get started.
Components You Need:
To successfully measure distance with Raspberry Pi and HC-SR04 sensor, you need,
- A Raspberry Pi 2 or 3 single board computer with Raspbian installed.
- A HC-SR04 ultrasonic sensor module.
- 3x10kΩ resistors.
- A breadboard.
- Some male to female connectors.
- Some male to male connectors.
I have written a dedicated article on installing Raspbian on Raspberry Pi, which you can check at https://linuxhint.com/install_raspbian_raspberry_pi/ if you need.
HC-SR04 Pinouts:
The HC-SR04 has 4 pins. VCC, TRIGGER, ECHO, GROUD.
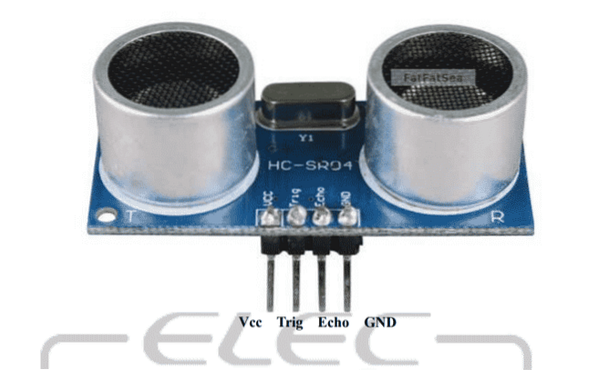
Fig1: HC-SR04 pinouts (https://www.mouser.com/ds/2/813/HCSR04-1022824.pdf)
The VCC pin should be connected to +5V pin of the Raspberry Pi, which is pin 2. The GROUND pin should be connected to the GND pin of the Raspberry Pi, which is pin 4.
The TRIGGER and ECHO pins should be connected to the GPIO pins of the Raspberry Pi. While, the TRIGGER pin can be directly connected to one of the GPIO pins of the Raspberry Pi, the ECHO pin needs a voltage divider circuit.
Circuit Diagram:
Connect the HC-SR04 ultrasonic sensor to your Raspberry Pi as follows:
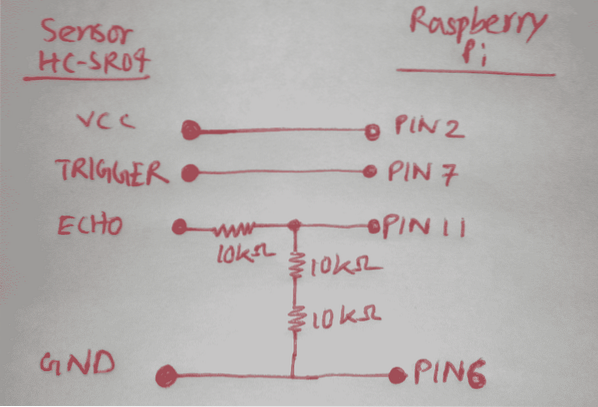
Fig2: HC-SR04 ultrasonic sensor connected to Raspberry Pi.
Once everything is connected, this is how it looks like:
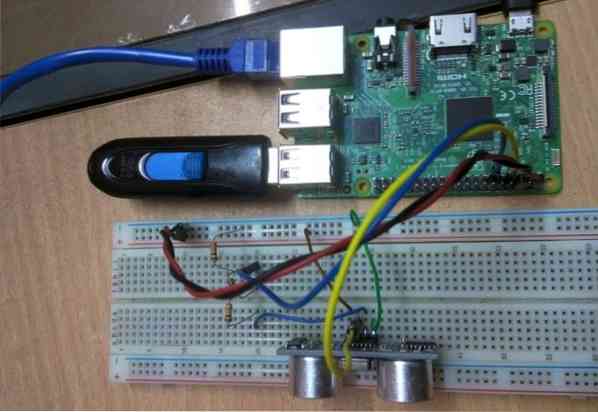
Fig3: HC-SR04 ultrasonic sensor connected to Raspberry Pi on breadboard.
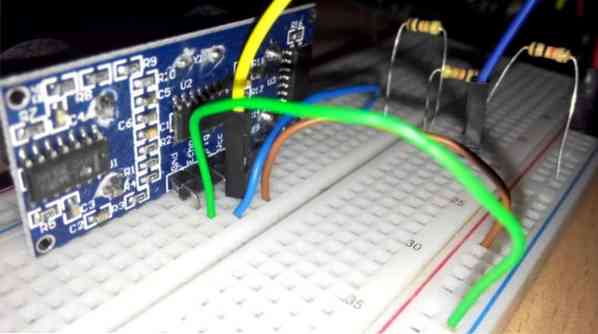
Fig4: HC-SR04 ultrasonic sensor connected to Raspberry Pi on breadboard.
Writing A Python Program for Measuring Distance with HC-SR04:
First, connect to your Raspberry Pi using VNC or SSH. Then, open a new file (let's say distance.py) and type in the following lines of codes:
Here, line 1 imports the raspberry pi GPIO library.
Line 2 imports the time library.
Inside the try block, the actually code for measuring the distance using HC-SR04 is written.
The finally block is used to clean up the GPIO pins with GPIO.cleanup() method when the program exits.
Inside the try block, on line 5, GPIO.setmode(GPIO.BOARD) is used to make defining pins easier. Now, you can reference pins by physical numbers as it is on the Raspberry Pi board.
On line 7 and 8, pinTrigger is set to 7 and pinEcho is set to 11. The TRIGGER pin of HC-SR04 is connected to the pin 7, and ECHO pin of HC-SR04 is connected to the pin 11 of the Rapsberry Pi. Both of these are GPIO pins.
On line 10, pinTrigger is setup for OUTPUT using GPIO.setup() method.
On line 11, pinEcho is setup for INPUT using GPIO.setup() method.
Lines 13-17 are used for resetting pinTrigger (by setting it to logic 0) and setting the pinTrigger to logic 1 for 10ms and then to logic 0. In 10ms, the HC-SR04 sensor sends 8 40KHz pulse.
Lines 19-24 are used to measure the time it takes for the 40KHz pulses to be reflected to an object and back to the HC-SR04 sensor.
On line 25, the distance is measured using the formula,
Distance = delta time * velocity (340M/S) / 2
=> Distance = delta time * (170M/S)
I calculated the distance in centimeters instead of meters, just to be precise. I calculated distance is also rounded to 2 decimal places.
Finally, on line 27, the result is printed. That's it, very simple.
Now, run the Python script with the following command:
$ python3 distance.pyAs you can see, the distance measured is 8.40 cm.
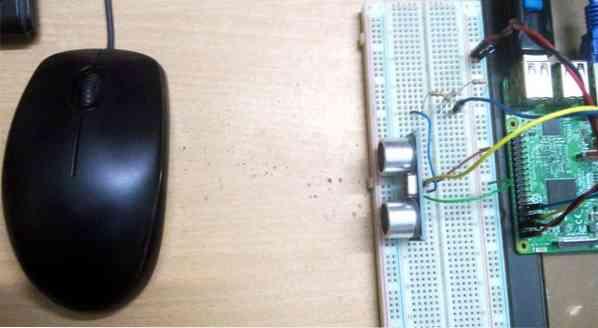
Fig5: object placed at about 8.40cm away from the sensor.
I moved to object a little bit farther, the distance measured is 21.81cm. So, it's working as expected.
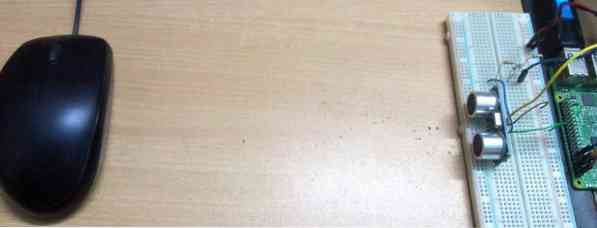
Fig6: object placed at about 21.81 cm away from the sensor.
So that's how you measure distance with Raspberry Pi using the HC-SR04 ultrasonic sensor. See the code for distance.py below:
import RPi.GPIO as GPIOimport time
try:
GPIO.setmode(GPIO.BOARD)
pinTrigger = 7
pinEcho = 11
GPIO.setup(pinTrigger, GPIO.OUT)
GPIO.setup(pinEcho, GPIO.IN)
GPIO.output(pinTrigger, GPIO.LOW)
GPIO.output(pinTrigger, GPIO.HIGH)
time.sleep(0.00001)
GPIO.output(pinTrigger, GPIO.LOW)
while GPIO.input(pinEcho)==0:
pulseStartTime = time.time()
while GPIO.input(pinEcho)==1:
pulseEndTime = time.time()
pulseDuration = pulseEndTime - pulseStartTime
distance = round(pulseDuration * 17150, 2)
print("Distance: %.2f cm" % (distance))
finally:
GPIO.cleanup()